The following cheat sheet for commonly used Python Turtle commands will get you up and running with Python Turtle quickly. Turtle is a fun program that dates all the way back to the 1960s when Seymour Papert and his colleagues at MIT created the programming language LOGO which could control a robot turtle with a physical pen in it. Today Turtle Graphics are most often associated with the Python programming language.
Depending on the language you are working with, you must recall a fair share of syntax, commands, and functions. Even if you work with code every day, it’s information that’s easy to forget. That’s exactly what our cheat sheets are for. The Python cheat sheets we provide contain common syntaxes and data properties.
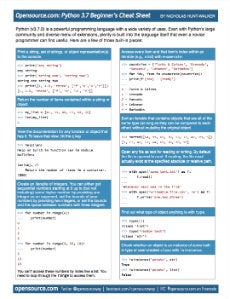
Python Turtle Commands
import turtle | Import the turtle library |
turtle_obj = Turtle() | Creates a new Turtle object and opens its window. |
turtle_obj.home() | Moves turtle_obj to the center of the window and then points turtle_obj east. |
turtle_obj.up() | Raises turtle_obj’s pen from the drawing surface. |
turtle_obj.down() | Lowers turtle_obj’s pen to the drawing surface. |
turtle_obj.setheading(degrees) | Points turtle_obj in the indicated direction, which is specified in degrees. East is 0 degrees, north is 90 degrees, west is 180 degrees, and south is 270 degrees. |
turtle_obj.left(degrees) | Rotates turtle_obj to the left by the specified degrees. |
turtle_obj.right(degrees) | Rotates turtle_obj to the right by the specified degrees. |
turtle_obj.goto(x, y) | Moves turtle_obj to the specified position. |
turtle_obj.forward(distance) | Moves turtle_obj the specified distance in the current direction. |
turtle_obj.backward(distance) | Moves turtle_obj the specified distance in the reverse direction. |
turtle_obj.pencolor(r, g, b) | Changes the pen color of turtle_obj to the specified RGB value |
turtle_obj.pencolor(string) | Changes the pen color of turtle_obj to the specified RGB value to the specified string, such as 'red'. Returns the current color of turtle_obj when the arguments are omitted. |
turtle_obj.fillcolor(r, g, b) | Changes the fill color of turtle_obj to the specified RGB value |
turtle_obj.fillcolor(string) | Changes the fill color of turtle_obj to the specified string, such as 'red'. Returns the current fill color of turtle_obj when the arguments are omitted. |
turtle_obj.begin_fill() | Enclose a set of turtle commands that will draw a filled shape using the current fill color. |
turtle_obj.end_fill() | Enclose a set of turtle commands that will draw a filled shape using the current fill color. |
turtle_obj.clear() | Erases all of the turtle’s drawings, without changing the turtle’s state. |
turtle_obj.width(pixels) | Changes the width of turtle_obj to the specified number of pixels. Returns turtle_obj’s current width when the argument is omitted. |
turtle_obj.hideturtle() | Makes the turtle invisible. |
turtle_obj.showturtle() | Makes the turtle visible. |
turtle_obj.position() | Returns the current position (x, y) of turtle_obj. |
turtle_obj.heading() | Returns the current direction of turtle_obj. |
turtle_obj.isdown() | Returns True if turtle_obj’s pen is down or False otherwise. |
Python Turtle Tutorials

- A detailed Python cheat sheet with key data types, functions, and commands you should learn as a beginner. Free to download as PDF and PNG.
- Sequence Containers Indexing Base Types ©2012-2015 - Laurent Pointal Python 3 Cheat Sheet License Creative Commons Attribution 4 Latest version on: https://perso.
- Python Turtle Commands Cheat Sheet The following cheat sheet for commonly used Python Turtle commands will get you up and running with Python Turtle quickly.
List Of All Python Commands
Learn how to use all of the commands above in the following tutorials that have working Python example code and program results. Visual studio code download for macbook pro.
